Imagine walking into a bustling diner where everyone seems to know your name. That’s what happens when SQL injection occurs in a database system—it allows unauthorized access to sensitive information, just like that. Let’s break down what you need to know about this critical security threat.
İçerik
What is SQL Injection?
SQL injection (SQLi) is like a security breach where malicious users exploit software vulnerabilities. When you input your username and password into a login page, the system may mistakenly check if someone else exists with those details instead of verifying your account directly. This happens because of improper handling of user inputs in database queries.
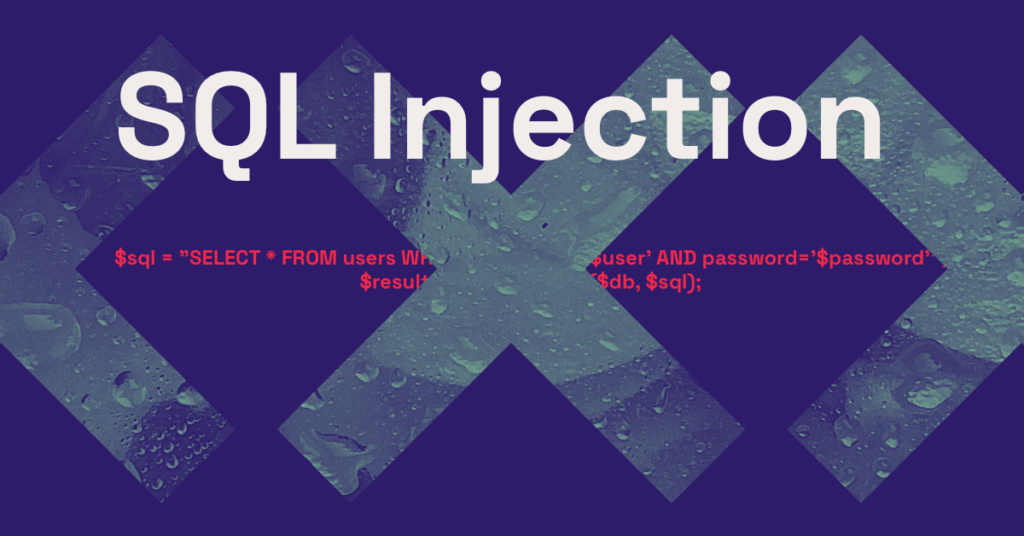
Why It’s a Problem
SQLi is dangerous because it lets attackers access data they shouldn’t. If a hacker can exploit a vulnerable system, they might gain control over sensitive info, leading to identity theft or financial fraud. It’s like leaving a back door open in your home—uninvited guests could walk right in.
How to Protect Against SQL Injection
Preventing SQLi requires careful handling of user data. Always use parameters when writing database queries. For example, instead of inserting user data directly into the database without validation, use prepared statements. In PHP, this might look like using mysqli Prepare Statements
to safely input values.
Here’s an example in PHP:
// Bad practice: vulnerable to SQL injection
$user = $_GET['user'];
$password = $_GET['pass'];
$sql = "SELECT * FROM users WHERE username='$user' AND password='$password'";
$result = mysqli_query($db, $sql);
In this code, user inputs are directly inserted into the query. Attackers can manipulate these inputs to gain access.
// Safer practice: using parameters
$user = $_GET['user'];
$password = $_GET['pass'];
$stmt = mysqli_prepare($db, "SELECT * FROM users WHERE username=? AND password= ?");
mysqli_stmt_bind_param($stmt, 'ss', $user, $password);
$result = mysqli_execute($db, $stmt);
Here, inputs are enclosed in ?
placeholders and bound securely. Attackers can’t easily inject malicious code.
Avoid Dynamic SQL
Dynamic SQL, where queries are built at runtime using user input, is risky. Instead of hardcoding all possible queries, use parameterized statements to limit what users can influence.
Use ORMs (Object-Relational Mapping)
Frameworks like Laravel or Symfony come with ORM tools that help build safe queries by abstracting database interactions. These tools can reduce the risk of SQLi by handling parameterization automatically.
Additional Tips
- Encrypt Communication: Use SSL/TLS to encrypt data in transit, making it harder for attackers to intercept.
- Security Headers: Implement headers like
Content Security Policy
to restrict what can be executed on the server side.
Stay Updated and Test
Regularly update your software and test for vulnerabilities. Tools like Burp Suite or SQLMap can help find weaknesses without needing technical expertise.
Conclusion
Preventing SQL injection is crucial for protecting sensitive data. By using parameters, avoiding dynamic SQL, and employing ORMs, you can safeguard your applications. Remember, staying informed and proactive is the best defense against these threats. Keep it safe online!